适用对象:品牌广告主和小店卖家
TikTok小店分析工具
让你获得10倍增长的TikTok数据
一站式TikTok数据分析平台
包含带货达人、视频素材、TikTok小店、爆款产品数据
注册免绑卡
为什么选择Tikstar?
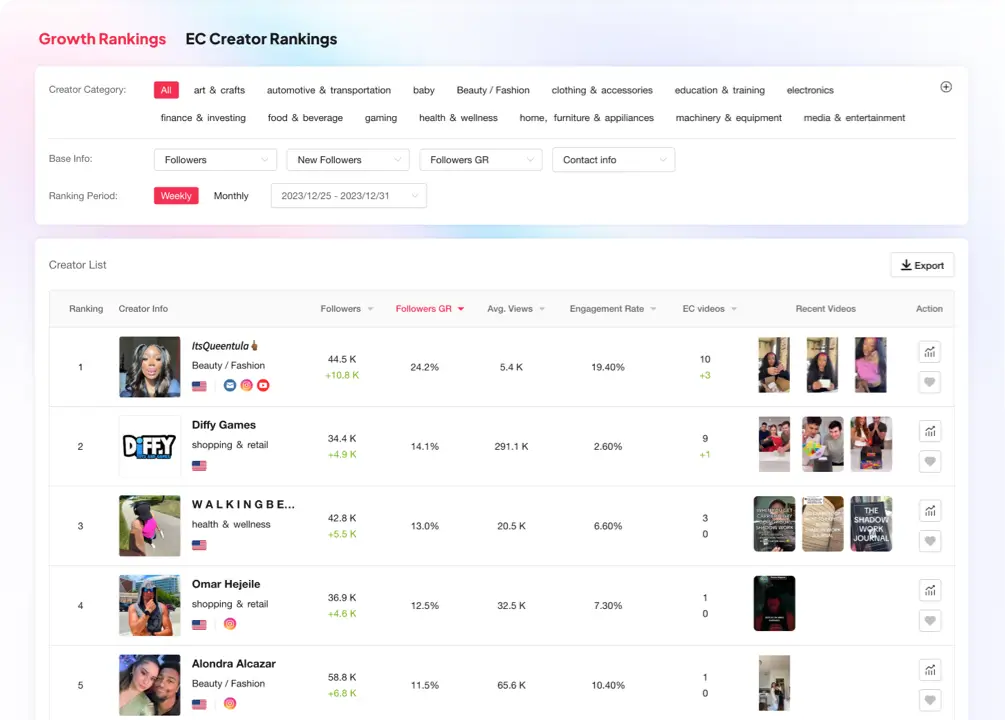
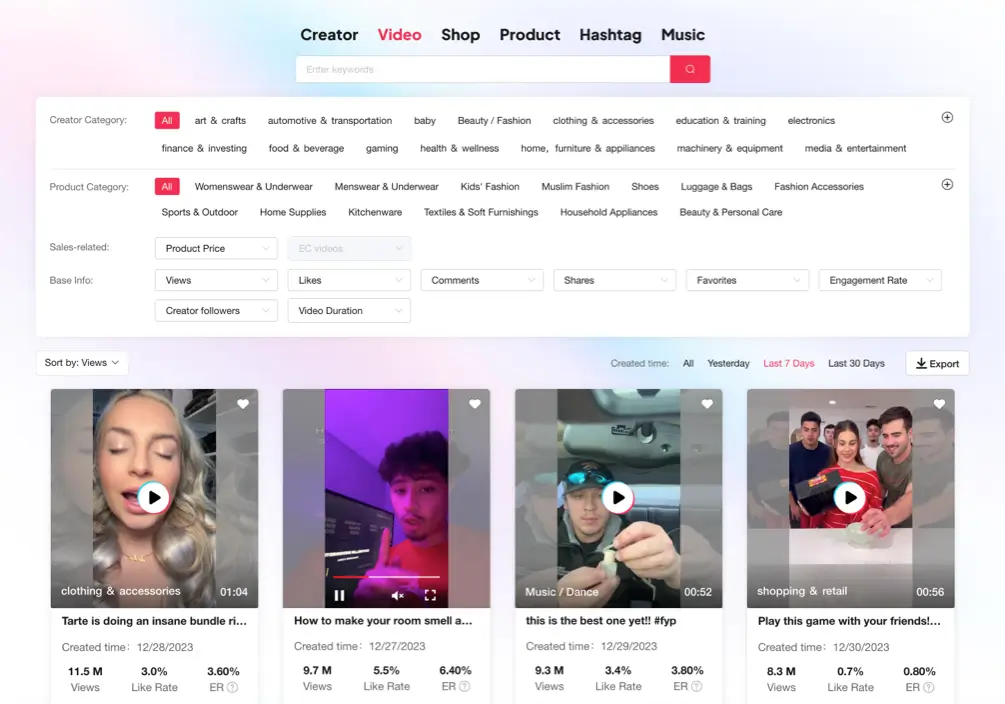
适用对象:TikTok达人和小店卖家
Tikstar协助你分析小店并发现爆款产品
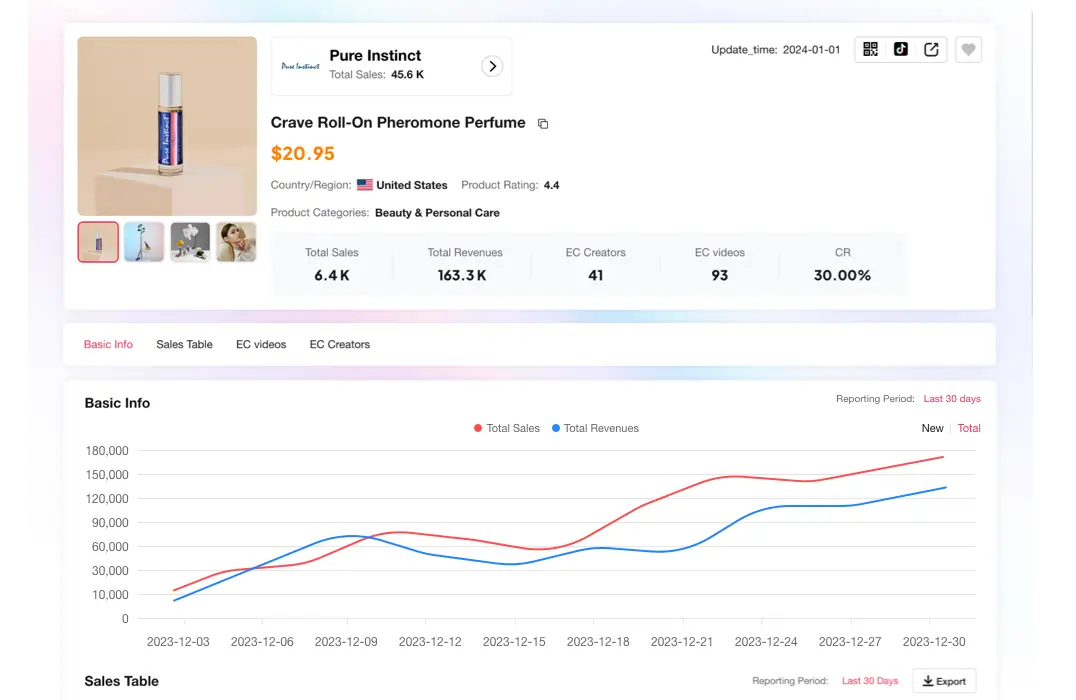
Tikstar还有哪些特色功能?
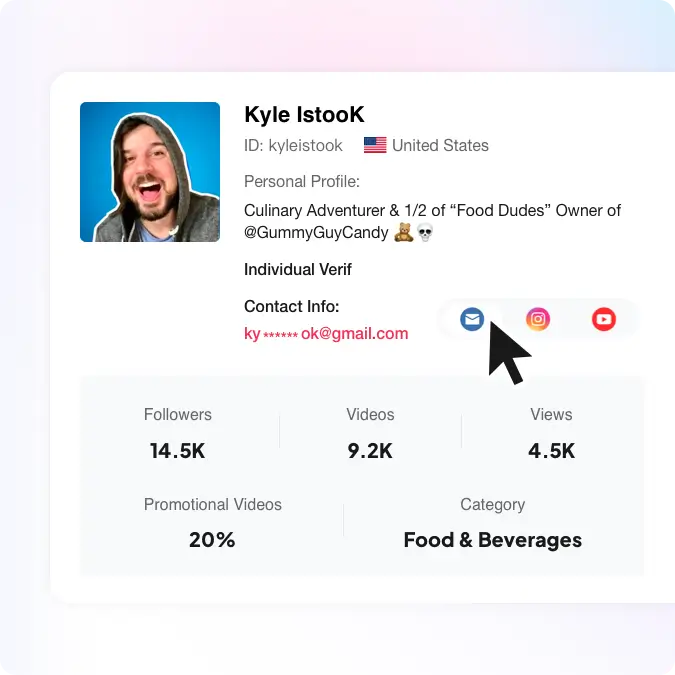
导出达人联系方式
提供达人的联系方式,包括邮箱、手机号、Ins、Twitter、YouTube等社媒账号信息。
开始试用支持全方位多维的筛选功能
提供各种高效便捷的筛选维度,诸如价格区间、平均播放量、新增销量和增长效率等。
免费试用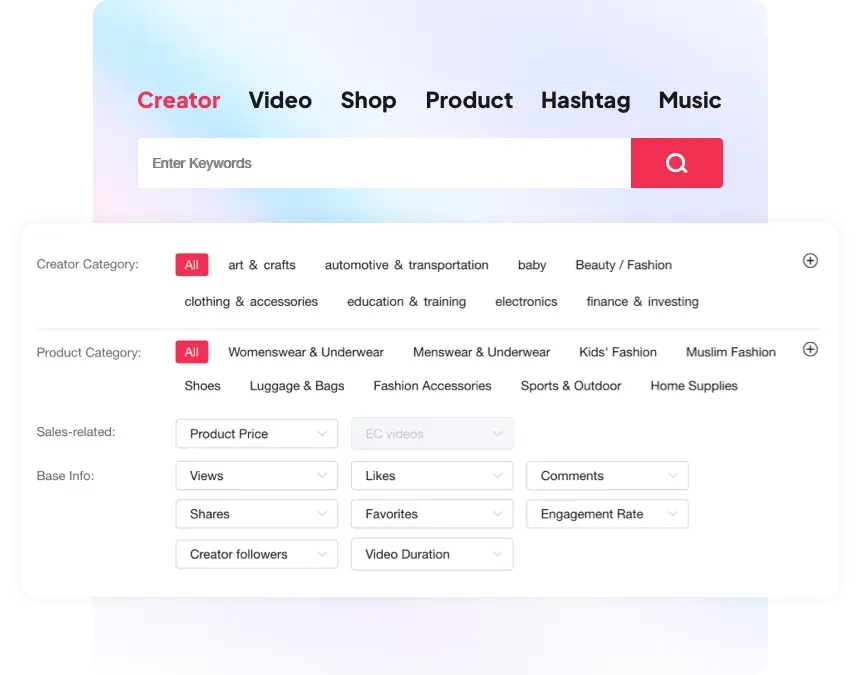